- Services
- Case Studies
- Technologies
- NextJs development
- Flutter development
- NodeJs development
- ReactJs development
- About
- Contact
- Tools
- Blogs
- FAQ
Enhancing App Security in Flutter: Best Practices
Protect your app and user data with proven techniques.
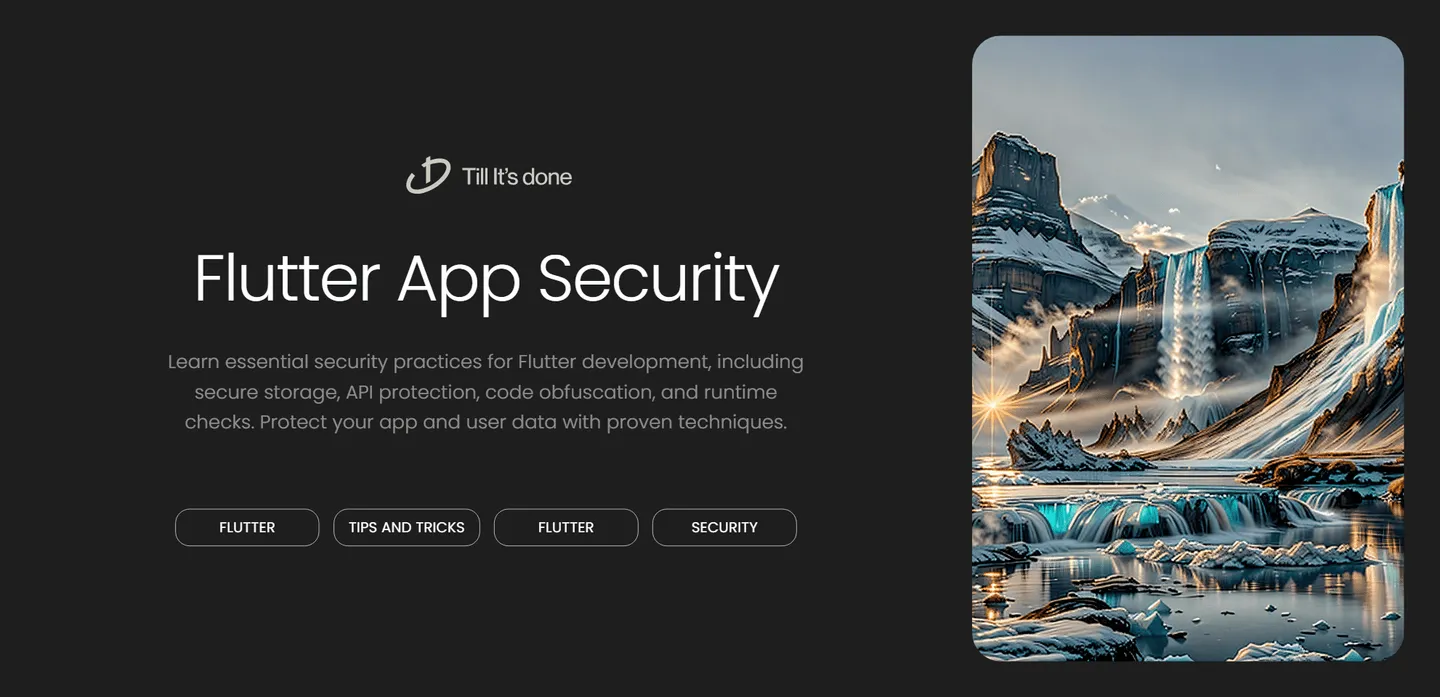
In today’s digital landscape, securing your Flutter applications isn’t just an option – it’s a necessity. As mobile apps handle increasingly sensitive data, implementing robust security measures has become crucial for developers. Let’s dive into some essential security best practices that will help you build more secure Flutter applications.
Secure Data Storage
When it comes to storing sensitive information locally, Flutter offers several secure options. Instead of using SharedPreferences for sensitive data, opt for Flutter Secure Storage. This package leverages platform-specific encryption: Keychain for iOS and EncryptedSharedPreferences for Android.
final storage = FlutterSecureStorage();await storage.write(key: 'auth_token', value: userToken);
API Security Best Practices
Always use HTTPS for network communications and implement certificate pinning to prevent man-in-the-middle attacks. Here’s how you can implement certificate pinning using the dio package:
void configureCertificatePinning() { dio.httpClientAdapter = IOHttpClientAdapter( createHttpClient: () { final client = HttpClient(); client.badCertificateCallback = (cert, host, port) => false; return client; }, );}
Code Obfuscation
Enable code obfuscation in your release builds to make reverse engineering more difficult. Add these settings to your build.gradle:
android { buildTypes { release { minifyEnabled true shrinkResources true proguardFiles getDefaultProguardFile('proguard-android.txt') } }}
Runtime Security Checks
Implement runtime security checks to detect potential security threats:
Future<bool> isDeviceSecure() async { if (Platform.isAndroid) { final isRooted = await RootDetection.isDeviceRooted(); final isEmulator = await EmulatorDetection.isEmulator(); return !isRooted && !isEmulator; } return true;}
Secure Authentication Patterns
Implement biometric authentication when available and ensure proper session management:
final localAuth = LocalAuthentication();final canCheckBiometrics = await localAuth.canCheckBiometrics;if (canCheckBiometrics) { final authenticated = await localAuth.authenticate( localizedReason: 'Please authenticate to access the app', );}
Data Encryption
For sensitive data that needs to be stored locally, implement encryption:
import 'package:encrypt/encrypt.dart';
final key = Key.fromSecureRandom(32);final iv = IV.fromSecureRandom(16);final encrypter = Encrypter(AES(key));
final encrypted = encrypter.encrypt('sensitive data', iv: iv);final decrypted = encrypter.decrypt(encrypted, iv: iv);
Remember to regularly update your dependencies, conduct security audits, and stay informed about the latest security vulnerabilities. Implementing these security measures from the start will help protect your users and their data from potential threats.
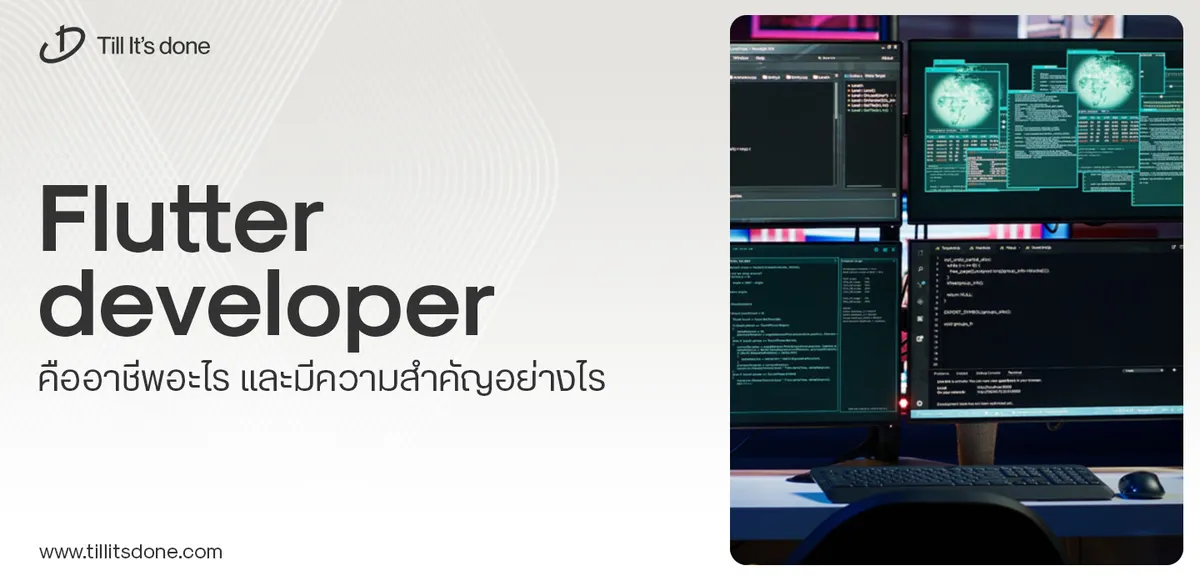
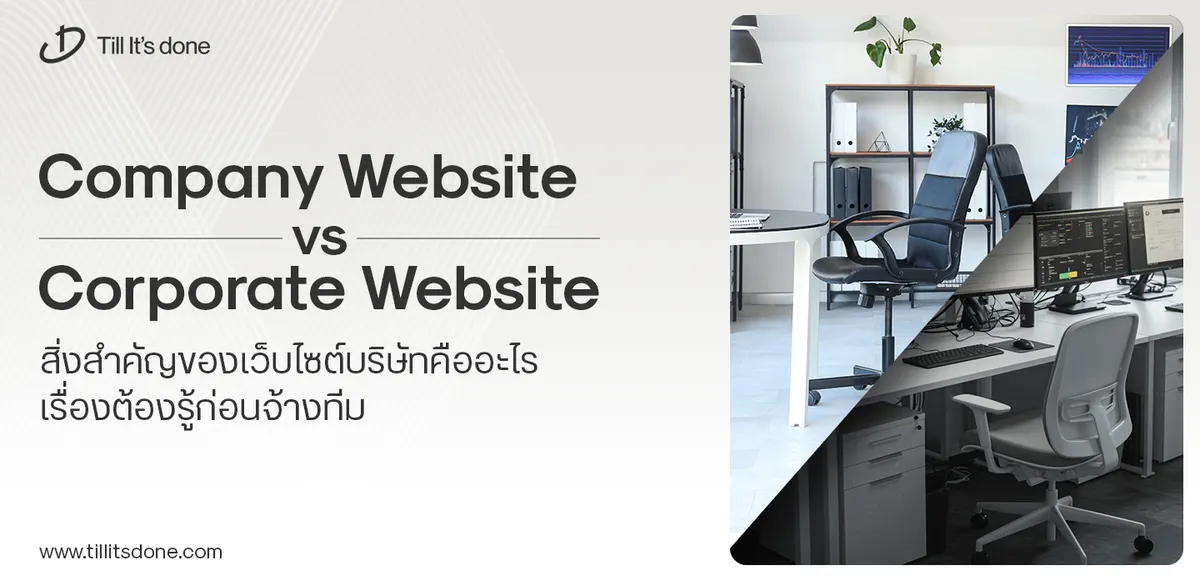
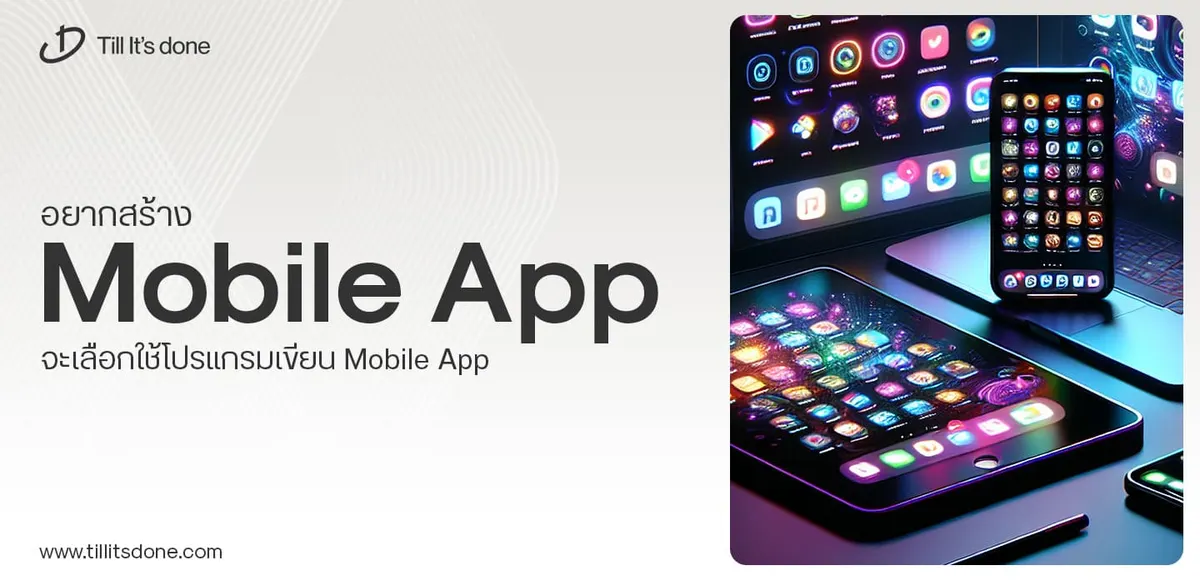
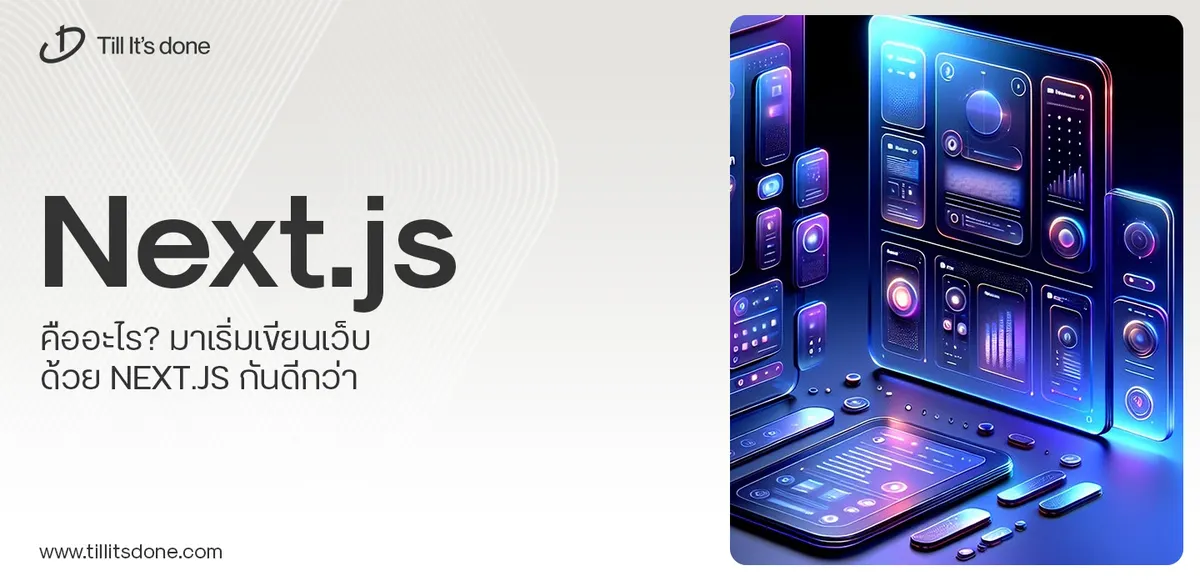
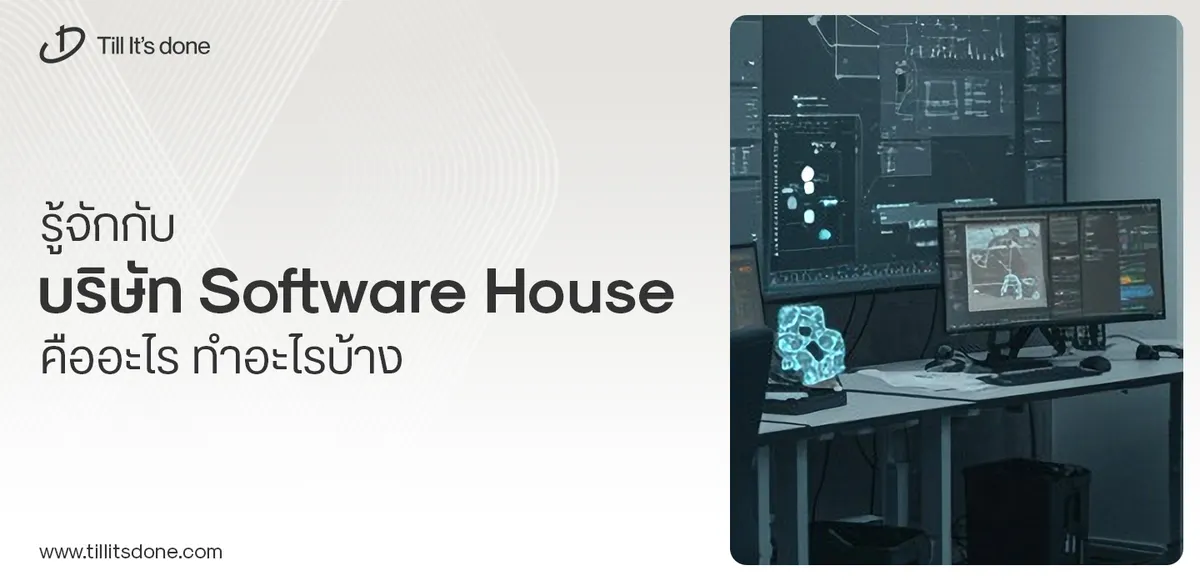
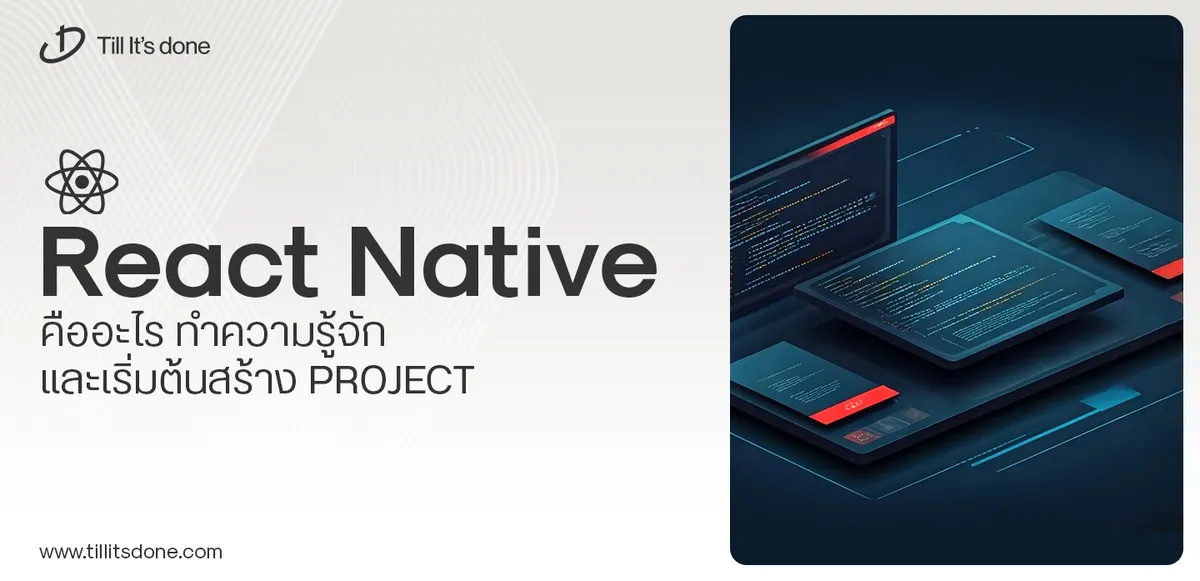
Talk with CEO
We'll be right here with you every step of the way.
We'll be here, prepared to commence this promising collaboration.
Whether you're curious about features, warranties, or shopping policies, we provide comprehensive answers to assist you.