- Services
- Case Studies
- Technologies
- NextJs development
- Flutter development
- NodeJs development
- ReactJs development
- About
- Contact
- Tools
- Blogs
- FAQ
Error Handling Guide for Express.js Apps
Discover best practices for custom error classes, async error handling, and global error middleware implementation.
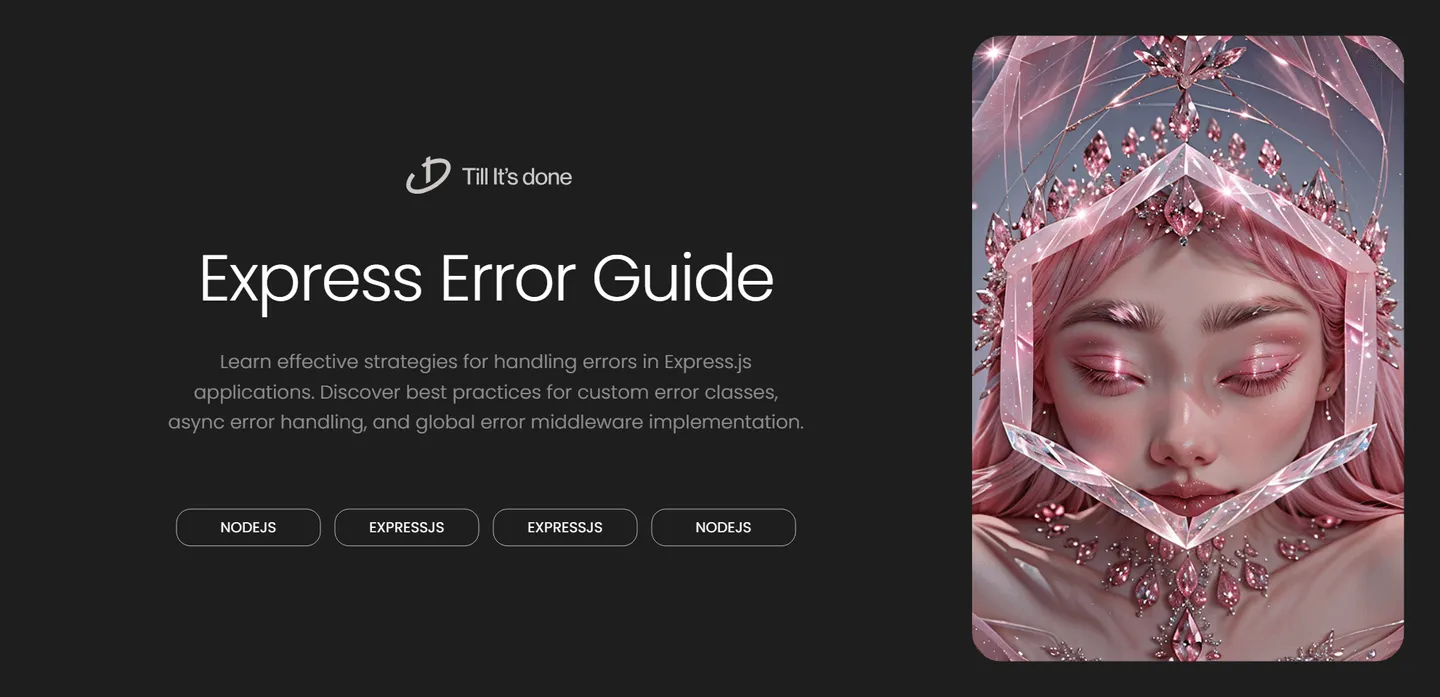
Handling Errors Gracefully in Express.js Applications
Error handling is one of those crucial aspects of building robust Node.js applications that often gets overlooked. In this post, I’ll share my experiences and best practices for handling errors effectively in Express.js applications.
Why Error Handling Matters
Think of error handling like wearing a seatbelt - you hope you never need it, but when things go wrong, you’re grateful it’s there. Poor error handling can lead to crashed servers, frustrated users, and countless hours debugging mysterious issues.
The Express Error Handling Basics
Let’s dive into how Express handles errors. By default, Express comes with a basic error handler, but we can do much better. Here’s my approach to structuring error handling:
1. Custom Error Classes
First, create custom error classes for different types of errors. This helps categorize and handle errors appropriately:
class ValidationError extends Error { constructor(message) { super(message); this.name = 'ValidationError'; this.status = 400; }}
2. Async Error Wrapper
One pattern I’ve found invaluable is creating a wrapper for async route handlers:
const asyncHandler = (fn) => (req, res, next) => { Promise.resolve(fn(req, res, next)).catch(next);};
3. Global Error Handler
The heart of our error handling strategy is the global error handler:
app.use((err, req, res, next) => { const status = err.status || 500; const message = err.message || 'Something went wrong';
res.status(status).json({ success: false, message, ...(process.env.NODE_ENV === 'development' && { stack: err.stack }) });});
Best Practices From Experience
- Always log errors appropriately
- Use different error status codes meaningfully
- Never expose sensitive error details in production
- Handle both synchronous and asynchronous errors
- Implement request validation early in the middleware chain
Monitoring and Debugging
Remember to set up proper monitoring for your error handling system. Tools like Winston or Pino for logging, combined with services like Sentry, can give you valuable insights into what’s going wrong in your application.
Remember, good error handling isn’t just about catching errors - it’s about providing meaningful feedback to both users and developers. Take the time to implement it properly, and your future self will thank you.
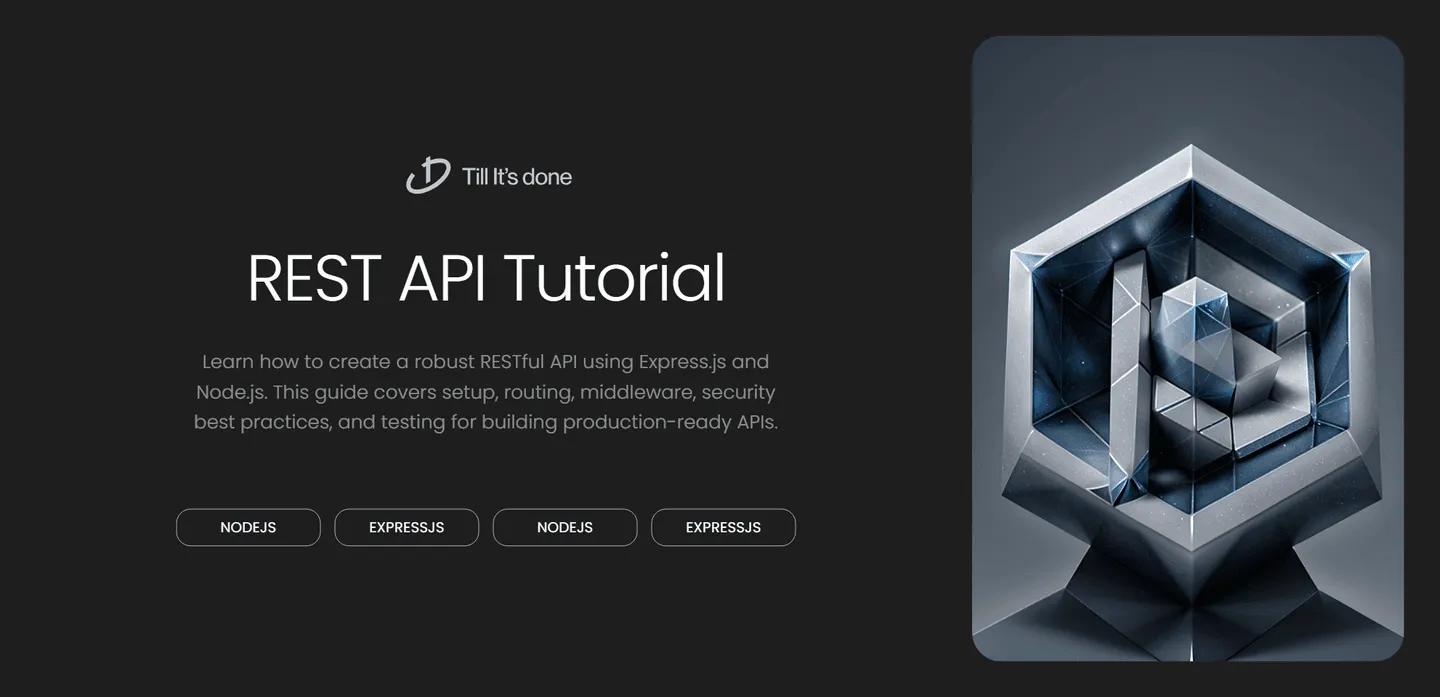
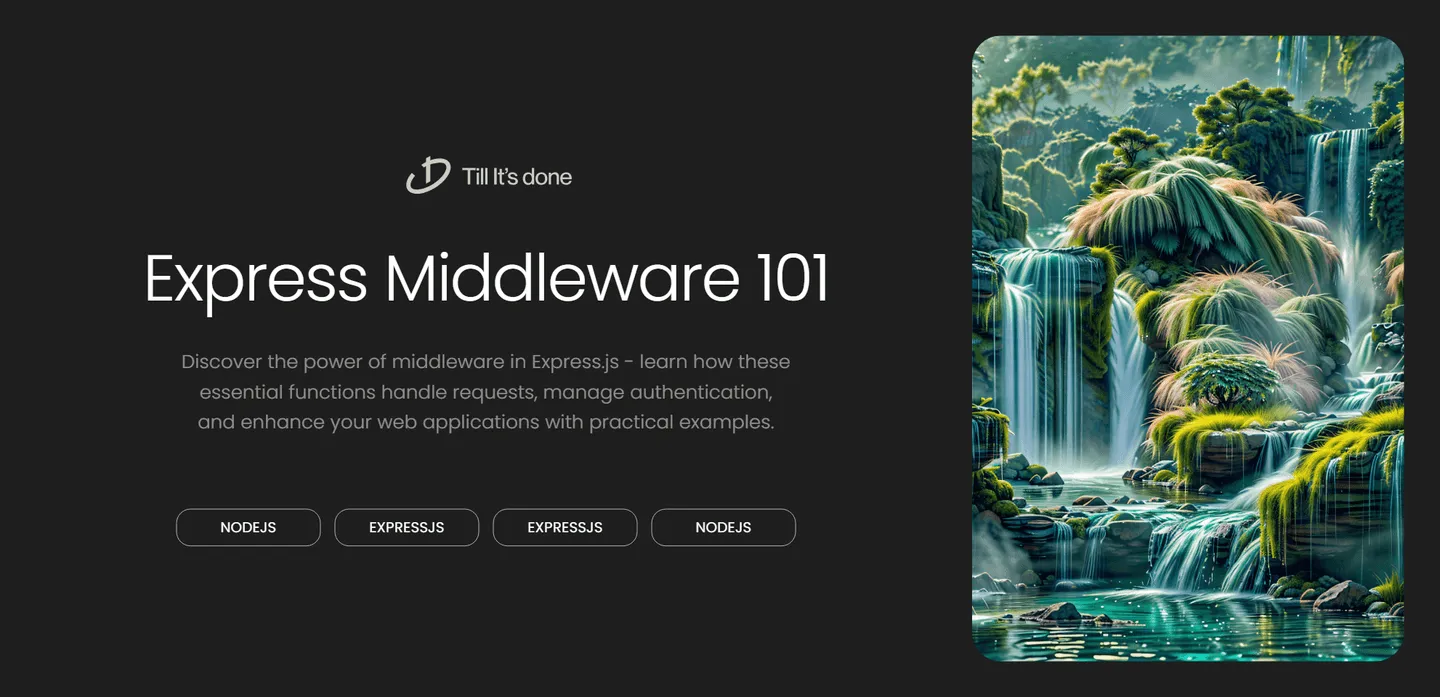
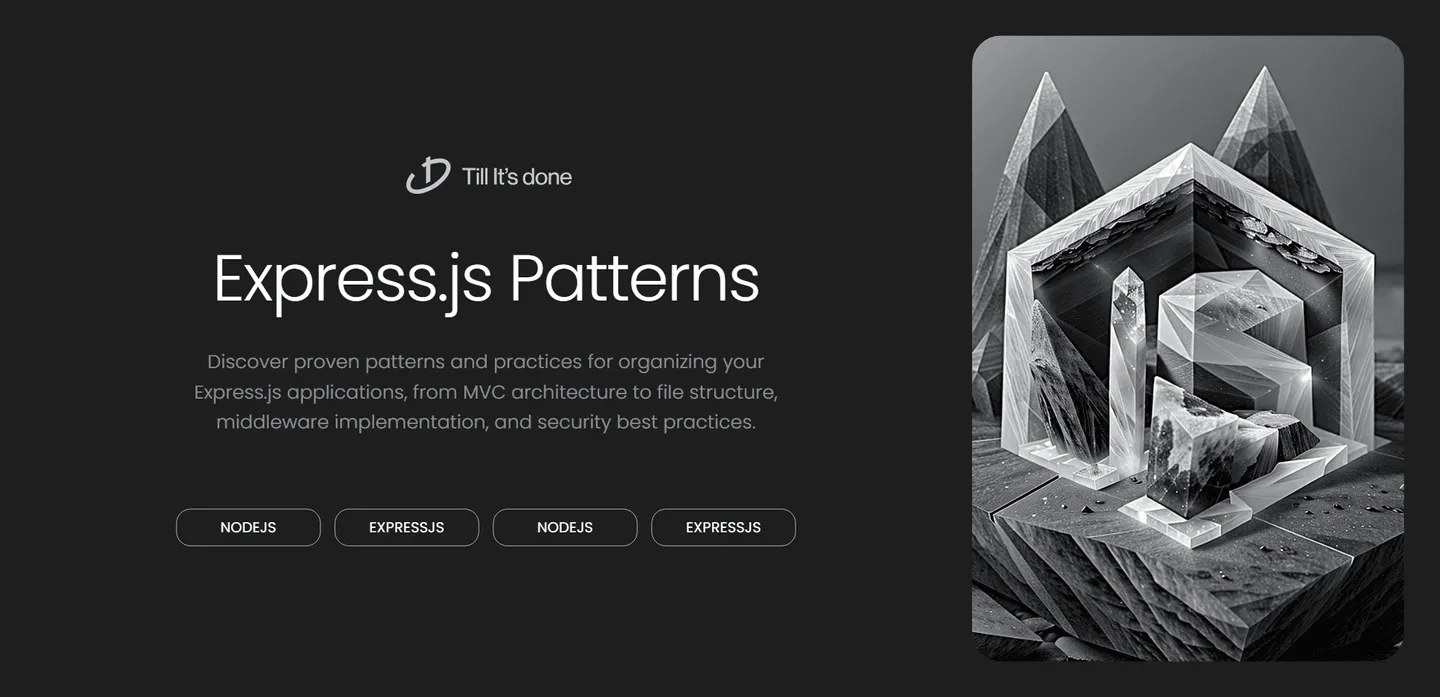
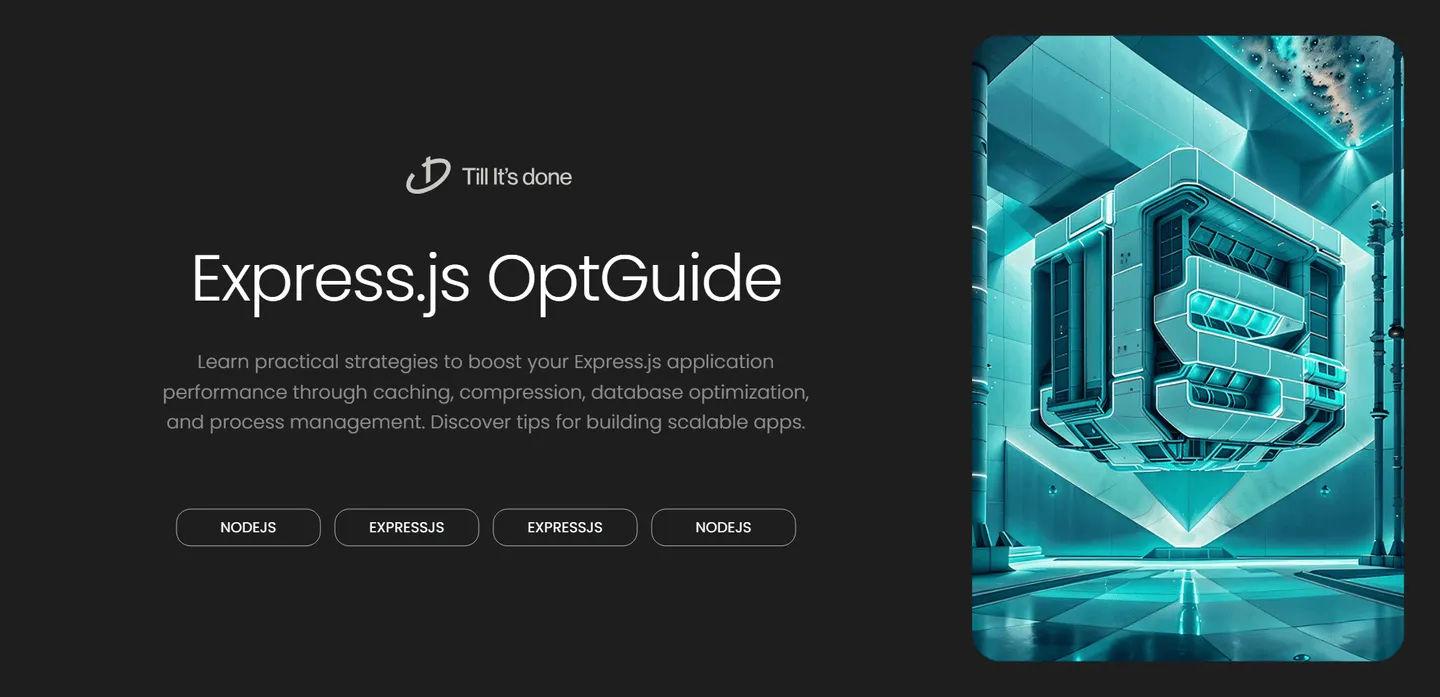
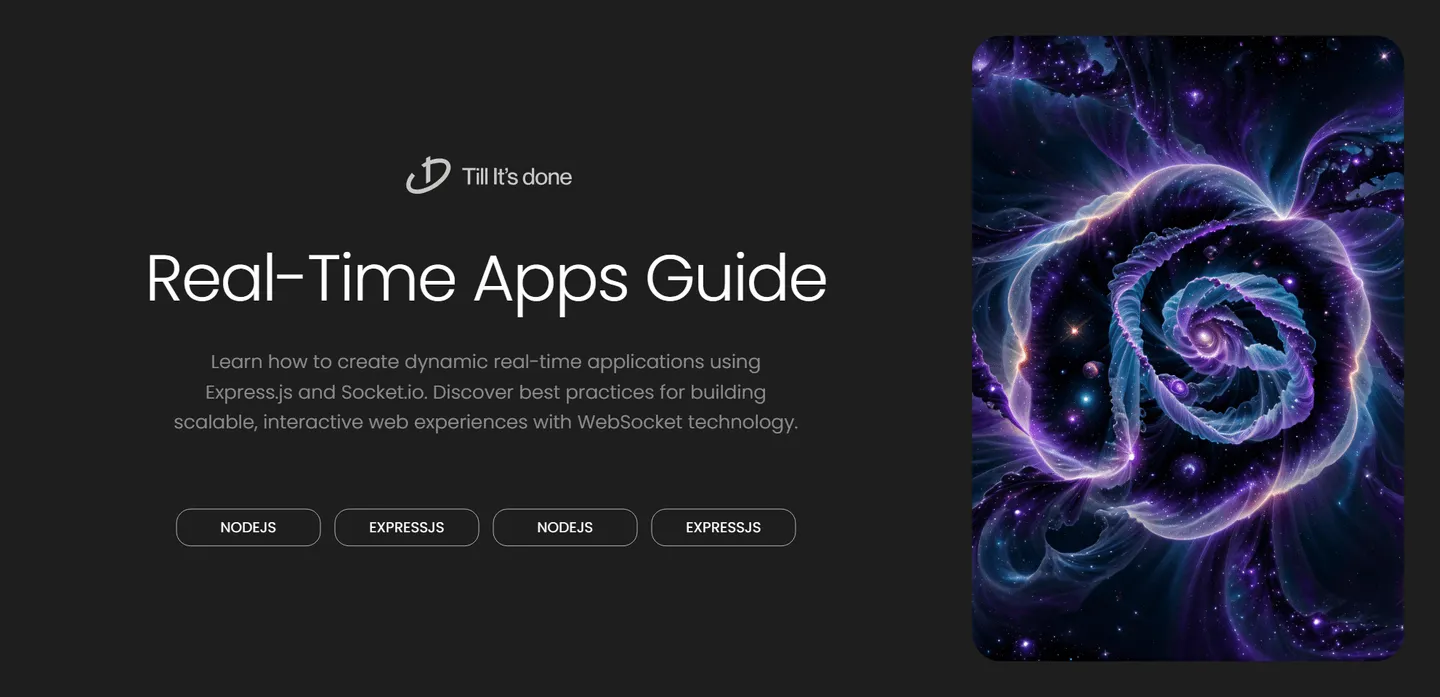

Talk with CEO
We'll be right here with you every step of the way.
We'll be here, prepared to commence this promising collaboration.
Whether you're curious about features, warranties, or shopping policies, we provide comprehensive answers to assist you.