- Services
- Case Studies
- Technologies
- NextJs development
- Flutter development
- NodeJs development
- ReactJs development
- About
- Contact
- Tools
- Blogs
- FAQ
Building CRUD Operations with Chi and Go
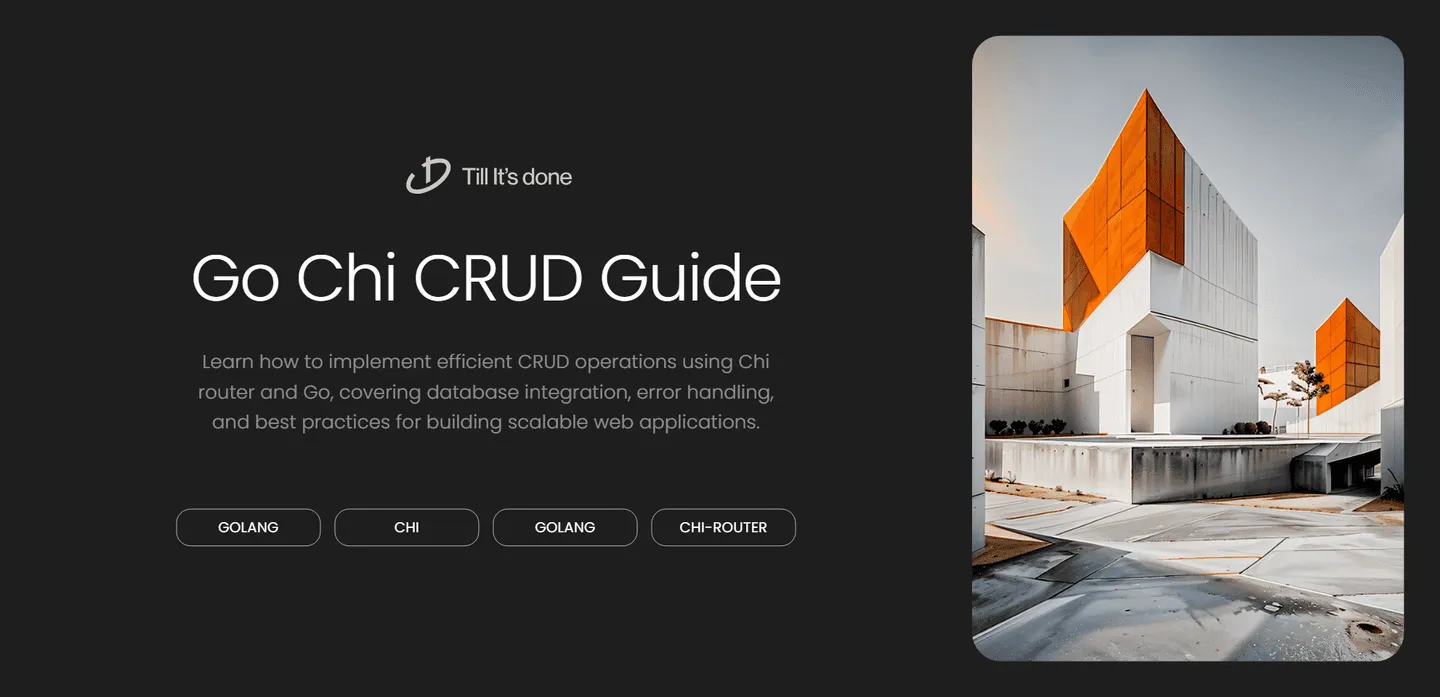
Building Robust CRUD Operations with Chi and Go: A Practical Guide
In today’s fast-paced development world, building efficient and scalable web applications is crucial. Go (Golang) has emerged as a powerful language for backend development, and when paired with the lightweight Chi router, it creates a formidable combination for building robust APIs. Let’s dive into creating a complete CRUD (Create, Read, Update, Delete) application using Chi and a database connection.
Setting Up Our Project Foundation
First, let’s structure our project properly. We’ll create a simple REST API for managing a book inventory system. This practical example will demonstrate real-world usage patterns and best practices.
package main
import ( "database/sql" "net/http" "github.com/go-chi/chi/v5" _ "github.com/lib/pq")
type Book struct { ID int `json:"id"` Title string `json:"title"` Author string `json:"author"` Year int `json:"year"`}
Implementing CRUD Operations
Let’s break down each operation and see how Chi’s routing capabilities make our code clean and maintainable. We’ll implement handlers for creating, reading, updating, and deleting books.
// Create a new bookfunc createBook(db *sql.DB) http.HandlerFunc { return func(w http.ResponseWriter, r *http.Request) { var book Book json.NewDecoder(r.Body).Decode(&book)
query := `INSERT INTO books (title, author, year) VALUES ($1, $2, $3) RETURNING id` err := db.QueryRow(query, book.Title, book.Author, book.Year).Scan(&book.ID)
if err != nil { http.Error(w, err.Error(), http.StatusInternalServerError) return }
json.NewEncoder(w).Encode(book) }}
The power of Chi shines through its middleware system. We can easily add authentication, logging, and error handling across our routes. Here’s how we set up our router with some basic middleware:
func setupRouter(db *sql.DB) *chi.Mux { r := chi.NewRouter()
r.Use(middleware.Logger) r.Use(middleware.Recoverer)
r.Route("/api/books", func(r chi.Router) { r.Post("/", createBook(db)) r.Get("/", getBooks(db)) r.Get("/{id}", getBook(db)) r.Put("/{id}", updateBook(db)) r.Delete("/{id}", deleteBook(db)) })
return r}
Error Handling and Best Practices
When working with databases, proper error handling is crucial. Here’s how we can implement a robust error handling system:
func handleDatabaseError(err error, w http.ResponseWriter) { switch { case err == sql.ErrNoRows: http.Error(w, "Resource not found", http.StatusNotFound) case err != nil: http.Error(w, "Internal server error", http.StatusInternalServerError) }}
Conclusion
Building CRUD operations with Chi and Go provides a clean, efficient, and maintainable solution for web applications. The combination of Go’s simplicity and Chi’s flexibility makes it an excellent choice for modern web development.
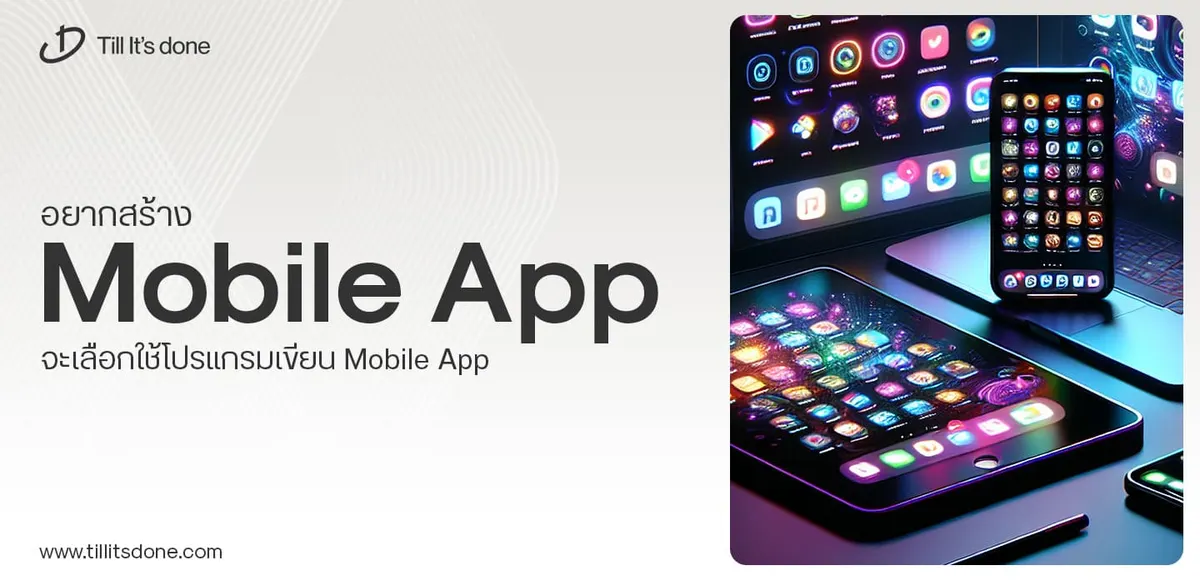
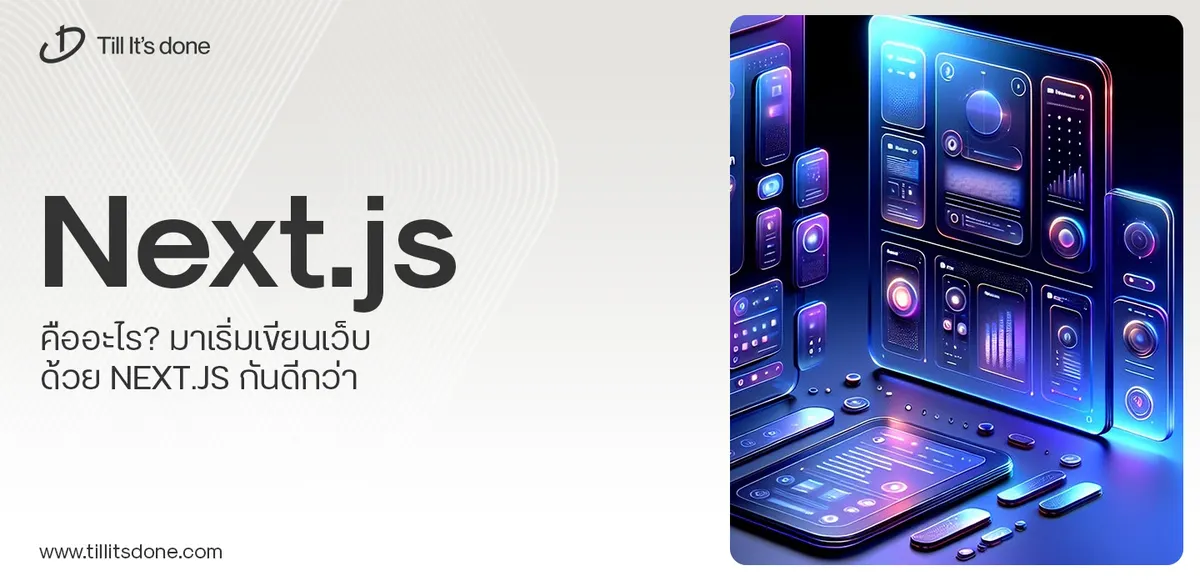
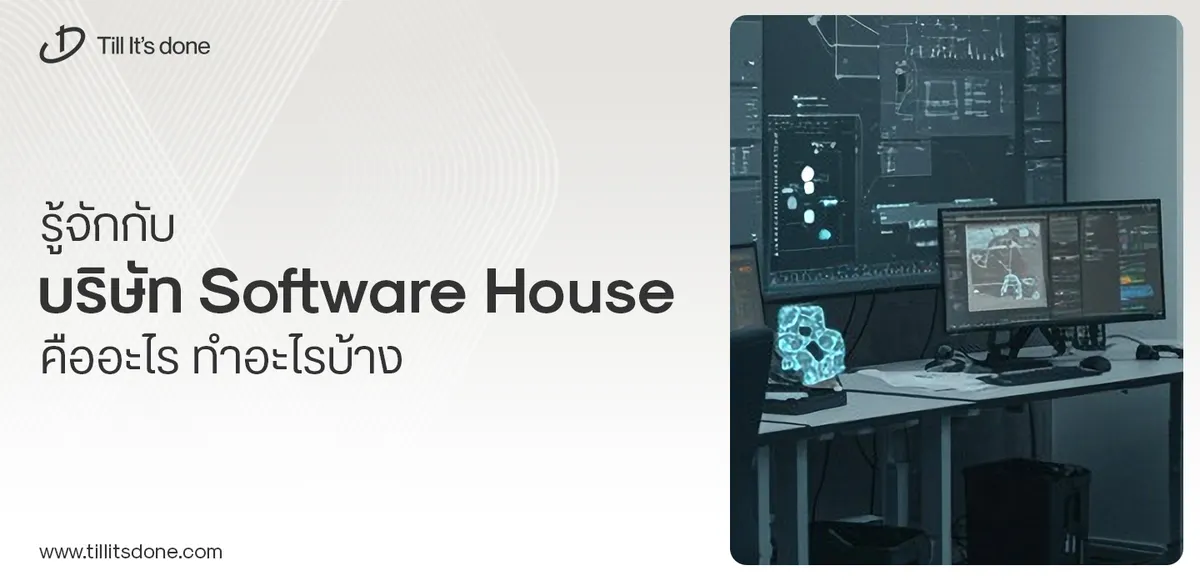
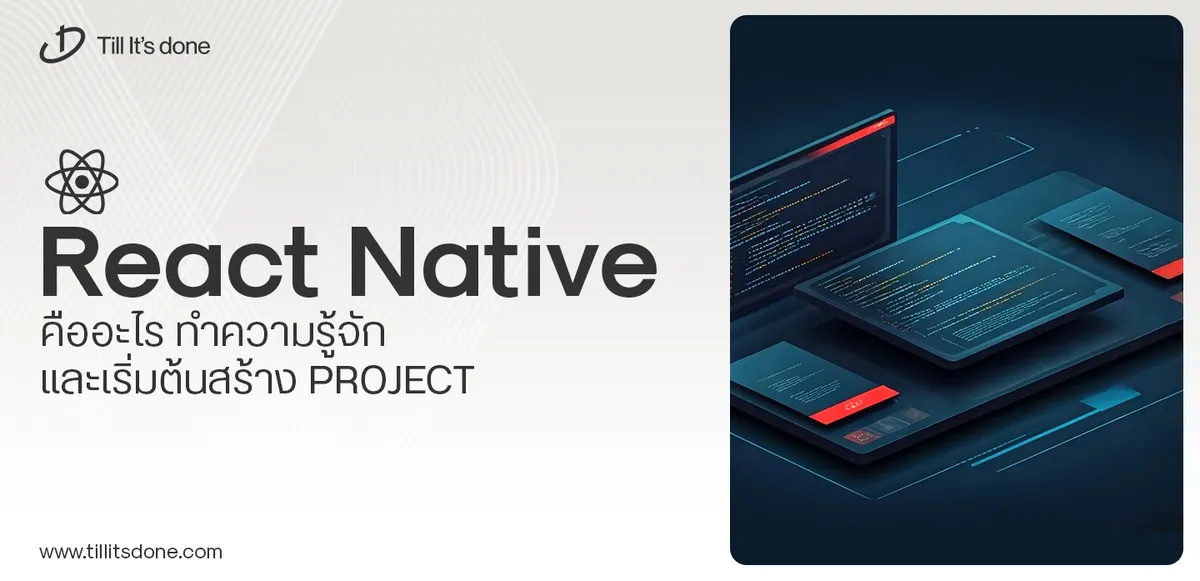
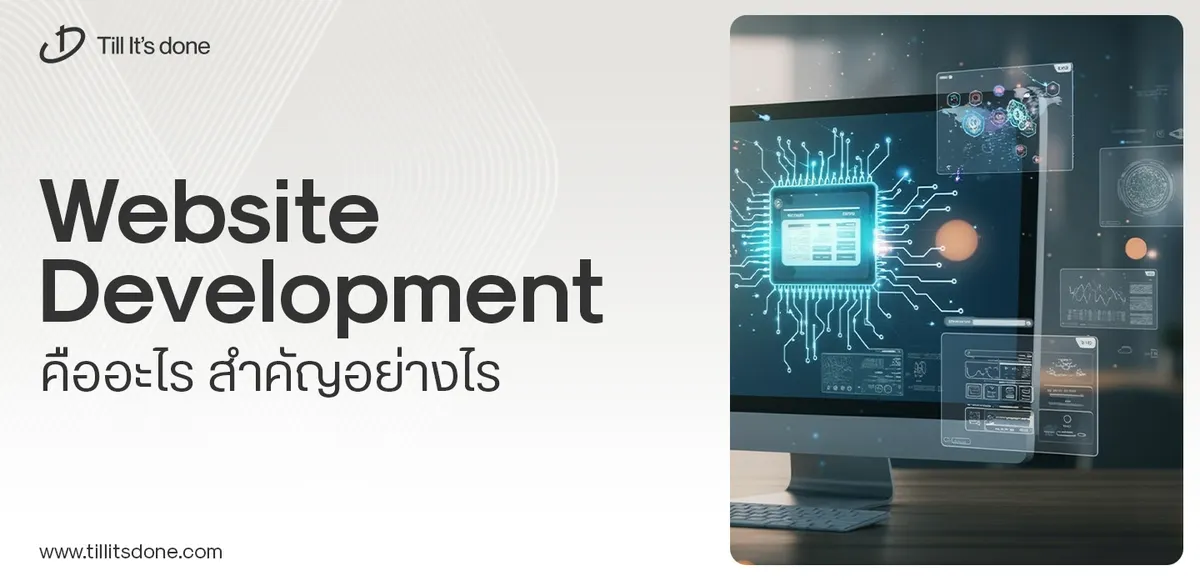
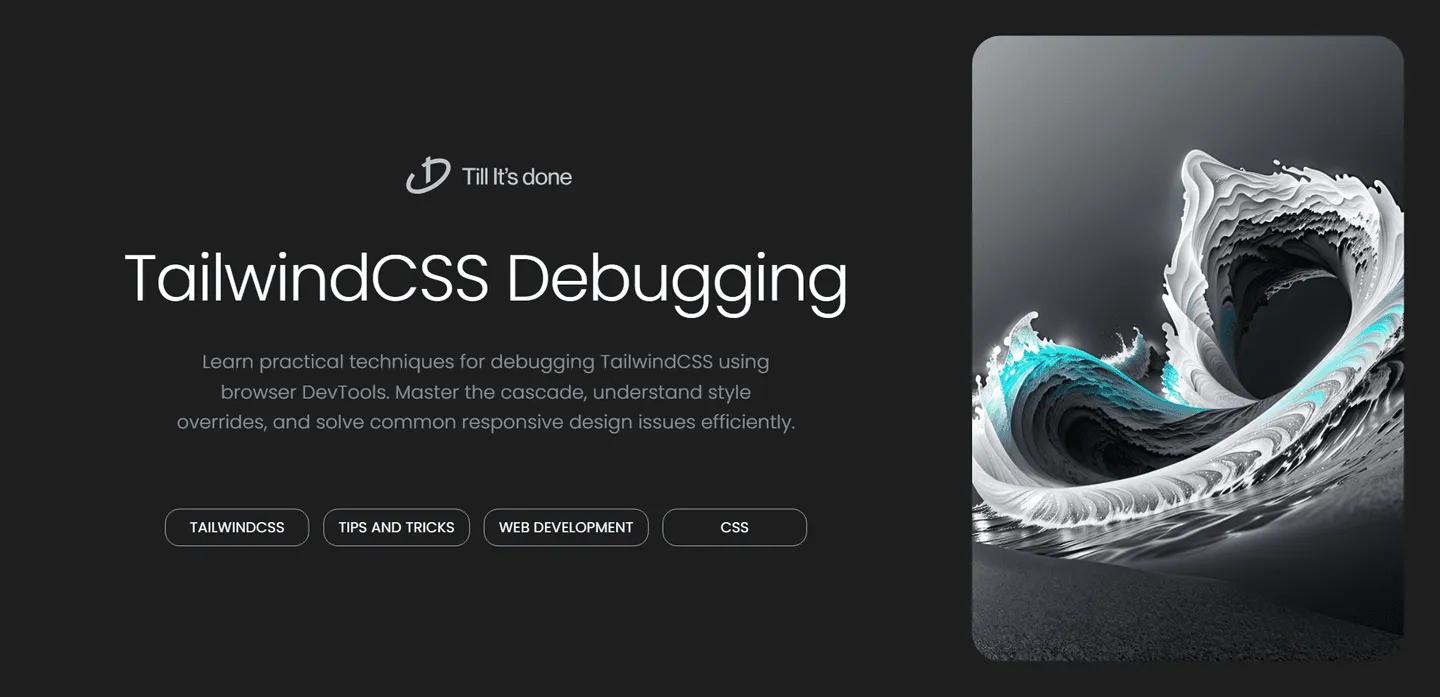
Talk with CEO
We'll be right here with you every step of the way.
We'll be here, prepared to commence this promising collaboration.
Whether you're curious about features, warranties, or shopping policies, we provide comprehensive answers to assist you.