- Services
- Case Studies
- Technologies
- NextJs development
- Flutter development
- NodeJs development
- ReactJs development
- About
- Contact
- Tools
- Blogs
- FAQ
How to Cancel Axios Requests in React Guide
Prevent memory leaks and handle race conditions like a pro.

How to Cancel Axios Requests in React
Have you ever encountered a situation where you needed to cancel an ongoing API request in your React application? Maybe the user navigated away from a page before the data finished loading, or perhaps they triggered a new search before the previous one completed. Today, let’s dive into how we can effectively manage and cancel Axios requests in React applications.
Why Cancel Requests?
Imagine you’re building a search feature that fetches results as users type. Each keystroke triggers a new API call, but what happens to all those pending requests? Without proper cancellation, they’ll continue running in the background, potentially leading to race conditions and unnecessary network traffic.
Understanding Axios Cancellation
Axios provides a powerful feature called Cancel Tokens that allows us to cancel pending requests. Let’s explore how to implement this in React.
Method 1: Using CancelToken.source()
The first approach involves creating a cancel token source. Here’s how you can implement it:
import axios from 'axios';import { useState, useEffect } from 'react';
function SearchComponent() { const [data, setData] = useState(null);
useEffect(() => { const source = axios.CancelToken.source();
const fetchData = async () => { try { const response = await axios.get('/api/search', { cancelToken: source.token }); setData(response.data); } catch (error) { if (axios.isCancel(error)) { console.log('Request cancelled:', error.message); } else { console.error('Error:', error); } } };
fetchData();
return () => { source.cancel('Operation cancelled by the user.'); }; }, []);
return <div>{/* Your component JSX */}</div>;}
Method 2: Using AbortController (Modern Approach)
The more modern approach leverages the AbortController API, which is now supported by Axios:
function SearchComponent() { useEffect(() => { const controller = new AbortController();
const fetchData = async () => { try { const response = await axios.get('/api/search', { signal: controller.signal }); // Handle response } catch (error) { if (axios.isCancel(error)) { console.log('Request cancelled'); } } };
fetchData();
return () => { controller.abort(); }; }, []);}
Best Practices and Tips
- Always clean up requests in useEffect’s cleanup function
- Handle cancellation errors gracefully
- Consider using a custom hook for reusable request cancellation
- Be mindful of race conditions in search implementations
- Use AbortController for modern applications
Conclusion
Properly managing API requests is crucial for building robust React applications. By implementing request cancellation, we can prevent memory leaks, avoid race conditions, and provide a better user experience.
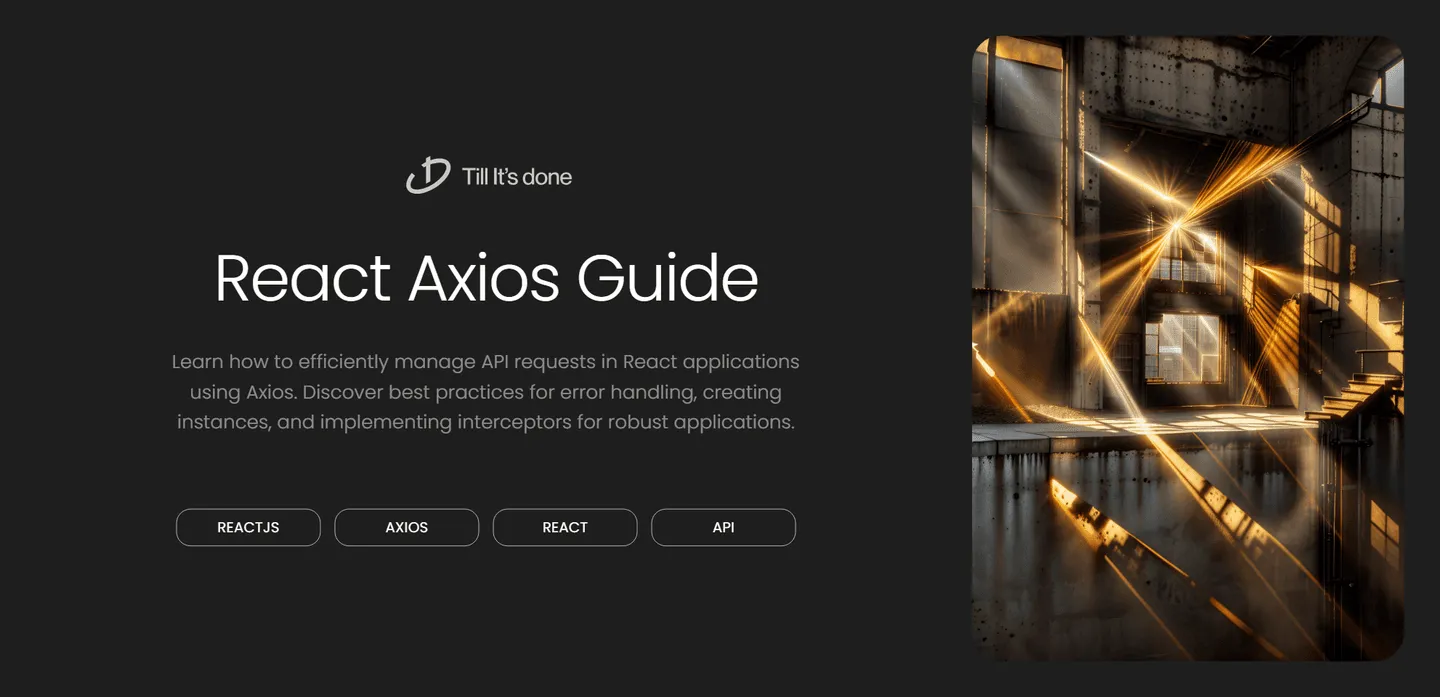



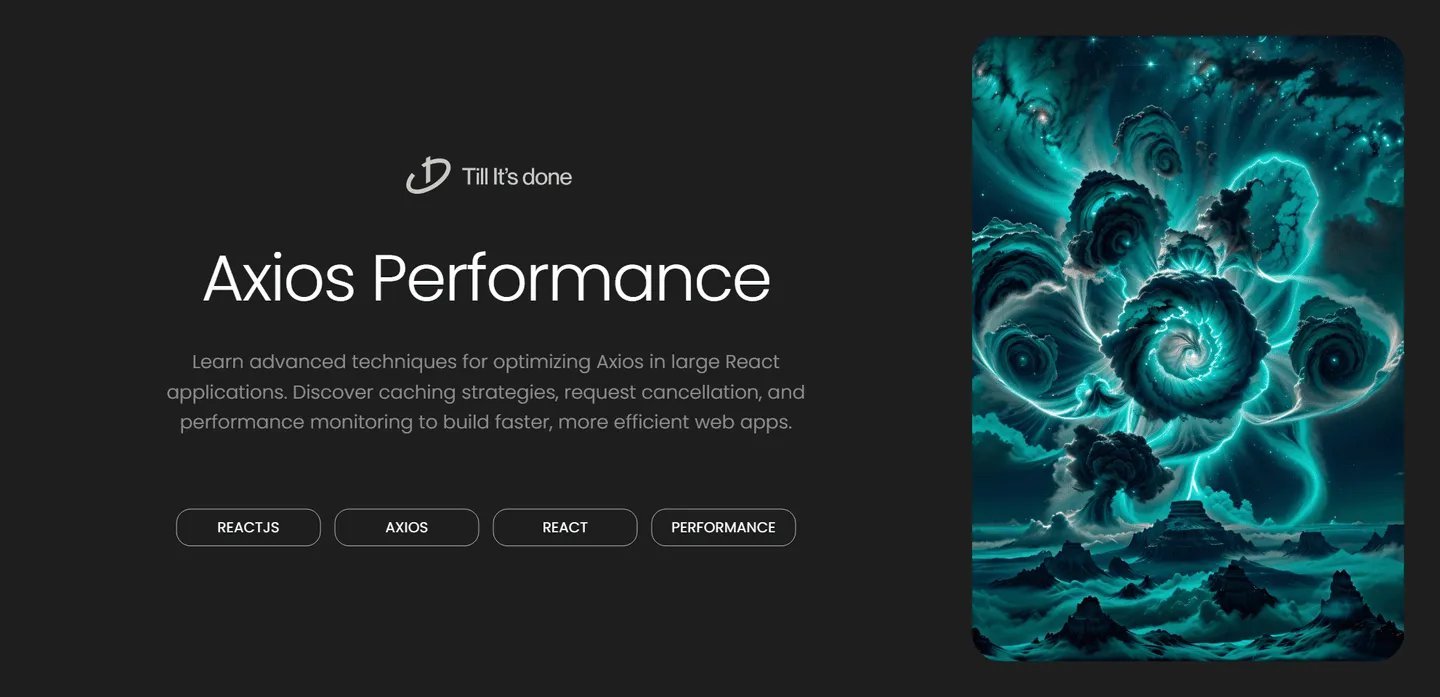

Talk with CEO
We'll be right here with you every step of the way.
We'll be here, prepared to commence this promising collaboration.
Whether you're curious about features, warranties, or shopping policies, we provide comprehensive answers to assist you.