- Services
- Case Studies
- Technologies
- NextJs development
- Flutter development
- NodeJs development
- ReactJs development
- About
- Contact
- Tools
- Blogs
- FAQ
Master Data Fetching with Axios and React Hooks
Discover best practices, error handling, and creating custom hooks for seamless data management in React applications.

Fetching Data from REST APIs with Axios and React Hooks
In today’s web development landscape, fetching and managing data from REST APIs is a crucial skill. Let’s dive into how we can effectively combine React Hooks with Axios to create clean, efficient data fetching solutions in our React applications.
Why Axios with React?
While the built-in fetch
API is capable, Axios brings several advantages to the table. It offers a more intuitive API, automatic JSON data transformation, and better error handling out of the box. When paired with React’s hooks, particularly useState
and useEffect
, we can create powerful data-fetching patterns.
Setting Up Your Project
First things first, let’s add Axios to our project. If you’re using npm:
npm install axios
Or with yarn:
yarn add axios
Creating a Custom Hook for Data Fetching
Let’s create a reusable hook that we can use across our application:
import { useState, useEffect } from 'react';import axios from 'axios';
const useDataFetching = (url) => { const [data, setData] = useState(null); const [loading, setLoading] = useState(true); const [error, setError] = useState(null);
useEffect(() => { const fetchData = async () => { try { const response = await axios.get(url); setData(response.data); setError(null); } catch (err) { setError(err.message); setData(null); } finally { setLoading(false); } };
fetchData(); }, [url]);
return { data, loading, error };};
export default useDataFetching;
Implementing POST Requests
Here’s how we can handle POST requests with error handling and loading states:
const submitData = async (data) => { try { setLoading(true); const response = await axios.post('https://api.example.com/data', data); setResponse(response.data); } catch (error) { setError(error.message); } finally { setLoading(false); }};
Best Practices and Tips
- Always include error handling
- Cancel requests when components unmount
- Use environment variables for API URLs
- Add loading states for better UX
- Implement request interceptors for auth tokens
Here’s a practical example combining these practices:
const UserProfile = () => { const { data, loading, error } = useDataFetching('https://api.example.com/user');
if (loading) return <div>Loading...</div>; if (error) return <div>Error: {error}</div>;
return ( <div> <h1>{data.name}</h1> <p>{data.email}</p> </div> );};
Remember to handle rate limiting, implement caching strategies, and consider using React Query or SWR for more complex data-fetching scenarios.



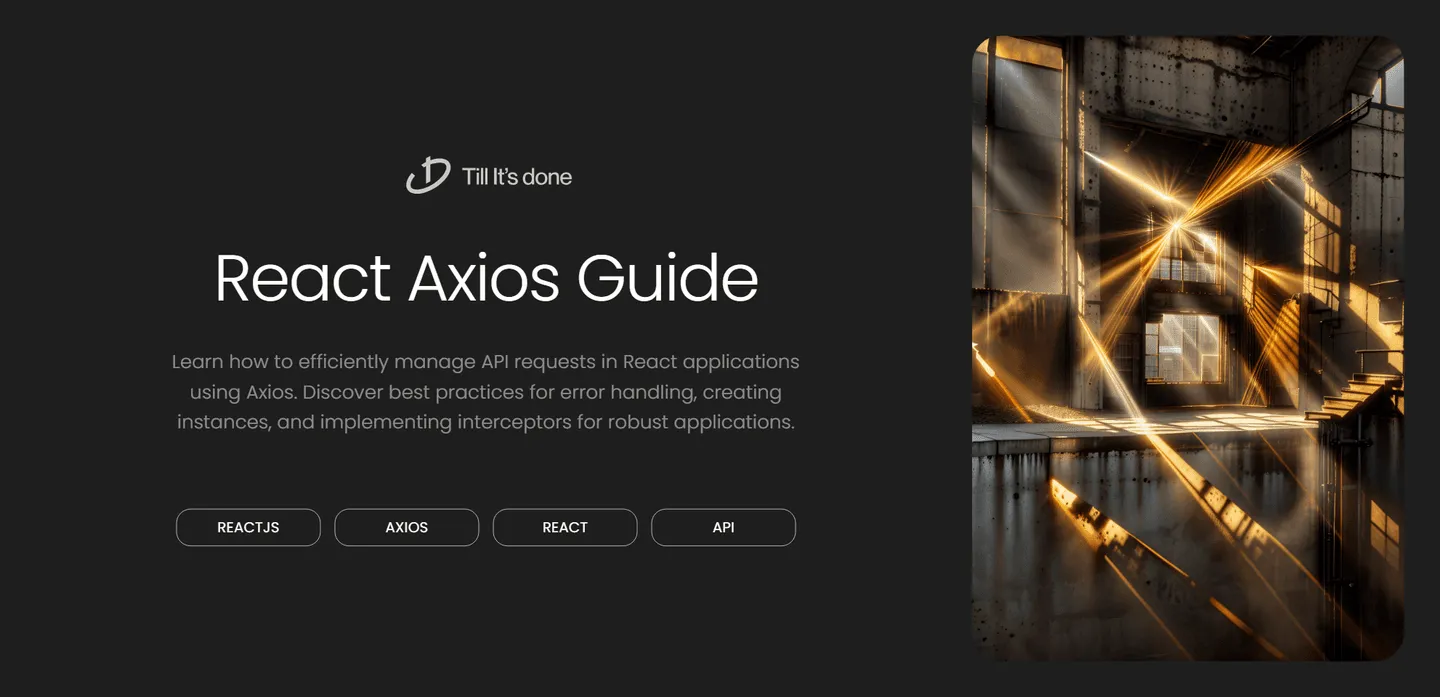

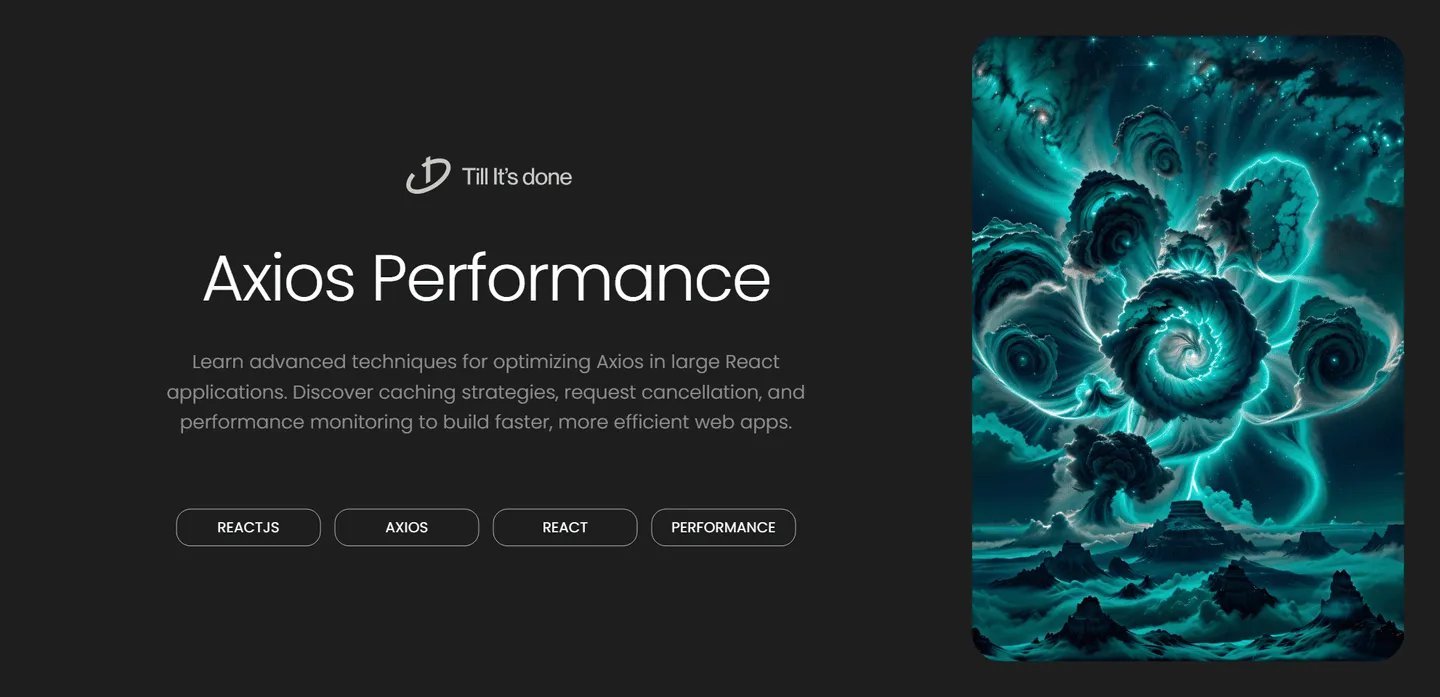
Talk with CEO
We'll be right here with you every step of the way.
We'll be here, prepared to commence this promising collaboration.
Whether you're curious about features, warranties, or shopping policies, we provide comprehensive answers to assist you.